开发一个基于区块链的信息共享平台
区块链技术已经成为信息共享和数据安全的重要工具。通过区块链,可以建立去中心化的、安全的信息共享平台,确保数据的透明性、不可篡改性和可验证性。下面我将为你提供一个基于区块链的信息共享平台的简要源码示例。
1. 智能合约
智能合约是区块链平台的核心组件,它定义了平台上的交易规则和逻辑。下面是一个简单的智能合约示例,用Solidity语言编写,部署在以太坊或类似的区块链上:
```solidity
pragma solidity ^0.8.0;
contract InformationSharingPlatform {
struct Information {
address owner;
string data;
}
mapping(uint => Information) public informationList;
uint public informationCount;
event InformationAdded(uint id, address owner, string data);
function addInformation(string memory _data) public {
informationCount ;
informationList[informationCount] = Information(msg.sender, _data);
emit InformationAdded(informationCount, msg.sender, _data);
}
}
```
这个智能合约定义了一个简单的信息共享平台,用户可以通过addInformation函数添加信息,并在事件InformationAdded中触发信息添加事件。
2. 前端界面
用户可以通过前端界面与智能合约交互。下面是一个简单的网页界面示例,使用Web3.js库连接到智能合约:
```html
window.addEventListener('load', async () => {
if (window.ethereum) {
window.web3 = new Web3(window.ethereum);
await window.ethereum.enable();
} else if (window.web3) {
window.web3 = new Web3(window.web3.currentProvider);
} else {
console.log('NonEthereum browser detected. You should consider trying MetaMask!');
}
// Contract ABI
const abi = [
// Contract ABI here
];
// Contract address
const address = 'CONTRACT_ADDRESS';
// Contract instance
const contract = new web3.eth.Contract(abi, address);
// Add Information
document.getElementById('addInformation').addEventListener('submit', async (event) => {
event.preventDefault();
const data = document.getElementById('data').value;
await contract.methods.addInformation(data).send({ from: web3.eth.defaultAccount });
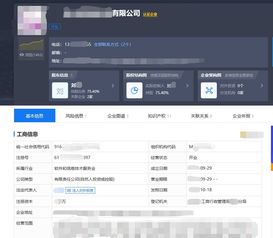
});
});
Information Sharing Platform